Measurement Protocol in Google Analytics 4
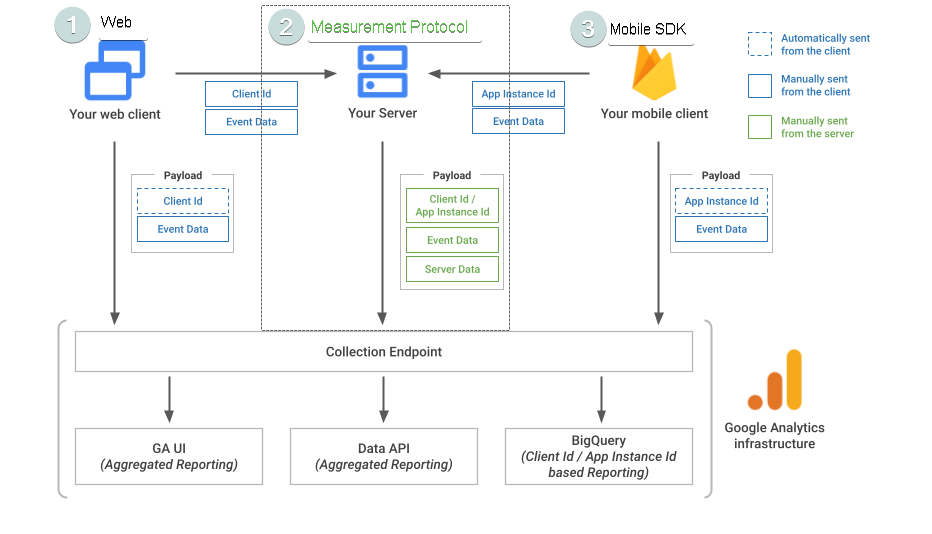
Google Analytics 4 provides three primary methods of data collection. The measurement protocol is one of the three methods.
In this article, we will explore what is measurement protocol and a practical example in python on how can we use a programming language to send analytics data to google analytics 4.
What is Measurement Protocol
The measurement protocol is a feature of Google Analytics 4 that allows developers to send data to Google Analytics from any device. This is different from “google tag” based data collection which works only for web/HTML applications. Measurement protocol works by allowing applications to send events directly to Google Analytics servers via HTTP requests.
Practical use cases
A few of the use cases where measurement protocol can help in collecting analytics data:
- Tracking IOT devices e.g. Reporting on home automation.
- Tracking offline conversions e.g. Reports directly from the POS system in retail shops.
- Omni-Channel Tracking e.g. Integrating with CRM data or other system data to create a 360 view of the customer.
Architecture
Official documentation for measurement protocol has a very detailed diagram of architecture. I will use that diagram and explain it in simple terms here.
The diagram above shows a complete architecture of how google analytics collects data using 3 supported technologies. The rectangular box around number 2 indicates the role and place of measurement protocol in augmenting the data collection.
There are 2 important terms used to explain data transport from clients ( e.g. Web or mobile ) to the google analytics server.
- Payload
- Collection Endpoint
Payload
Payload is used to refer to the data that is being transmitted as part of a request. For example, in an HTTP POST request payload may include a file, form data, an image, or just some text. In the context of google analytics payload is data sent to google analytics servers to track user behavior and events. It consists of parameters that google tag generates and google server expects. Upon receiving the payload data, the server processes it and reports it in the google analytics interface.
Collection endpoint
The collection endpoint is the url that routes the requests to Google Analytics servers using measurement protocol. It is responsible for receiving and processing the received data. It takes the form of :
https://www.google-analytics.com/mp/collect?v=2&measurement_id=[MEASUREMENT_ID]&api_secret=[API_SECRET]
v=2: refers to measurement protocol version 2.
MEASUREMENT_ID: is the id of the stream which is collecting data.
API_SECRET: is generated from the stream mentioned above to authenticate the data requests.
Now, let us look at an actual example of sending a few measurement protocol requests from python. We will send the following hits
- Pageview event hit
- Event hit with User property
Step 1: Define the Endpoint URL
Getting Measurement ID
Measurement ID can be obtained from
Admin -> Data Streams -> Click on the Stream
Measurement ID is on the right most side, click on the copy icon to copy the id to the clipboard.
Getting API secret
In the same screen where you copied the measurement id, under the Events section, you can find Measurement Protocol API secrets. ( See above image). If you are generating it for the first time, Google will ask you to review and acknowledge the terms. Once done, click on the “Create” button and create a new API secret. You can choose any name, in this example, I have chosen MY-GA4.
Once done, you will see the new API secret and its value. Copy the value.
Creating Endpoint URL
We can complete our code for Endpoint URL.
# 1. We Define EndPoint URL and give it our Google Analytics 4 propertys' tream ID + API Secret. MEASUREMENT_ID = "G-JNBXVNZ28J" API_SECRET = "_5atIPeDTFydh-TFjJMlBQ" # Set the endpoint URL for the measurement protocol ENDPOINT_URL = f'https://www.google-analytics.com/mp/collect?v=2&measurement_id={MEASUREMENT_ID}&api_secret={API_SECRET}'
Step 2: Create the Payload
Payload is the data that is included in the request being sent to Google Analytics. It requires few parameters as mandatory.
Mandatory parameters
- Client ID
- Event Array(events [] array)
- –Event name(events[].name]
Google’s official documentation recommends that in order for auser to see the data in real-time report, we must send “session_id” and “engagement_time_msec” as part of the event parameters. However, at the time of this writing in March 2023, these parameters are not mandatory. The request goes successfully, as well as shows up in real-time report without these parameters.
In the code below, we will create a very simple page_view request to send to Google Analytics.
# 2. We create the Payload ( Event to be sent ) and declare the data for the measurement protocol hit payload = { "client_id": "1857116524.1675709798", "events": [ { "name": "page_view", "params": { "page_location": "http://googleanalytics4.co", } } ] }
Step 3: Send the Request
The final step is sending the request we just created. Since we are using measurement protocol version 2, we can post the request to the measurement protocol server. The server will always return a 2xx status code if the request was sent successfully. What that means is, even if the event payload was malformed, the server will respond with 200 in case of successful post. Event validation can be done separately, which we will explore in another article.
In the code below, we have used try, except block to make sure that if request is not sent successfully, we get an error for the same.
# 3. Send the measurement protocol hit using the Requests library
try:
response = requests.post(ENDPOINT_URL, data=json.dumps(payload))
response.raise_for_status() # Raise an error if the response has an HTTP error status code
print("Measurement protocol hit successfully sent.")
except requests.exceptions.HTTPError as e:
print(
f"Error sending measurement protocol hit. Status code: {e.response.status_code}. Error message: {e.response.text}")
except Exception as e:
print(f"Error sending measurement protocol hit: {e}")
Verifying the Hit
We can verify the hit from Google Analytics’ real-time report.
Looking at the Real-time report, we can see whether the event is appearing when we run the code. In this example, here’s the screenshot for the page_view event.
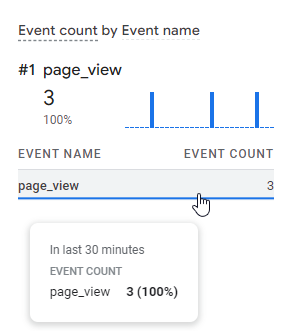
Since page_view can be triggered by several other users, you can choose an arbitrary name to identify the event. For example, if I change the event name in the above code to page_skew from page_view, my real-time report shows like this.
Summary
Measurement protocol is a feature that allows augmenting data collection from the web and mobile. It can be used to collect data from offline devices, retail POS, and several other hardware devices which have neither web browser nor mobile SDK support. A measurement protocol request consists of an end-point and payload. End-point is the URL of the measurement protocol where the request is being sent, the payload is the actual data being sent with the request. In this article, we sent a sample measurement protocol hit with minimum parameters using python and then verified it using real-time reports request in the Google Analytics server.
Responses