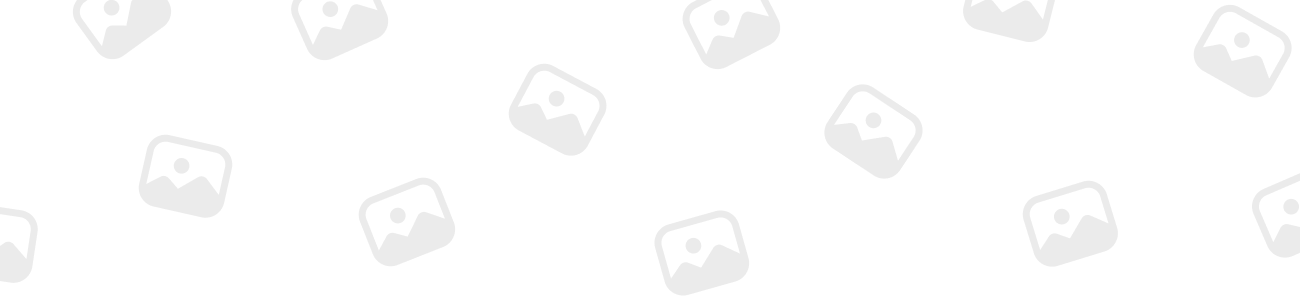
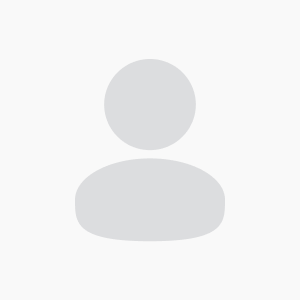
Elijah
Forum Replies Created
-
Elijah
Member6 July 2023 at 12:29 pm in reply to: Methods for sending access token to Google Analytics 4 Admin APIHere are the steps you need to follow:
First, you don’t need to worry about manually setting the access token. The Google PHP library has got you covered. It’s smart enough to fetch the token on its own. For this, you just need to point it to the service account JSON using the ‘GOOGLE_APPLICATION_CREDENTIALS’ environment variable.
So, let’s simplify your code a bit. Include the ‘vendor/autoload.php’ file and use the ‘AnalyticsAdminServiceClient’ class from the PHP library.
`php
require ‘vendor/autoload.php’;
use GoogleAnalyticsAdminV1alphaAnalyticsAdminServiceClient;
`
Next, use the ‘putenv’ function to set the path to your service account JSON file:
`php
putenv(‘GOOGLE_APPLICATION_CREDENTIALS=/path/to/your/service-account.json’);
`
Now, just create an instance of the ‘AnalyticsAdminServiceClient’ class. You don’t need to pass any access token here.
`php
$client = new AnalyticsAdminServiceClient();
`
Once the client is set, you can then fetch the list of accounts like so:
`php
$accounts = $client->listAccounts();
`
And finally, you can loop through these accounts and print their names:
`php
foreach ($accounts as $account) {
print ‘Found account: ‘ . $account->getName() . PHP_EOL;
}
`
And voilà! That’s pretty much it. Now you should be able to fetch the list of accounts without any issues. For more details, you can check the PHP Analytics Admin Samples on GitHub. Enjoy your coding experience!
-
Elijah
Member29 June 2023 at 10:49 pm in reply to: Extract Daily Ad Revenue from GA4-linked BigQuery Using Query LanguageIn Google Analytics 4 (GA4), ad revenue can be associated with multiple event names depending on the attribution system you’re using. However, two most common events where you’ll likely see ad revenue are “ad_impression” and “ad_click”. If you wish to sum up the ad revenue for each day, you need to locate the ad_revenue event parameter for these events.
Here’s a simple BigQuery query that might help:
`
SELECT
event_date,
SUM(event_params.value.double_value) as total_ad_revenue
FROM
your-project.dataset.events
,
UNNEST(event_params) AS event_params
WHERE
event_params.key = “ad_revenue”
GROUP BY
event_date
ORDER BY
event_date DESC
`
Regarding the LTV (Lifetime Value) Revenue returning NULL, it might be due to the fact that LTV metrics are not pre-aggregated or calculated in the raw event data that’s exported to BigQuery from GA4. The calculation and population of user-level LTV metrics usually happen within the Google Analytics interface, which means it might not be directly available in the BigQuery dataset.
-
Elijah
Member19 April 2023 at 1:54 am in reply to: Comparing User Numbers: GA4 Users Significantly Exceed Universal Analytics Users?The discrepancy could be due to GA4’s enhanced ability to track users across multiple devices and sessions as compared to UA’s more session-based approach. Also, consider the impact of timezone differences you may be based in and check your report on page views, sessions and total users. If the difference still persists, it might be due to the different definitions of ‘Users’ in GA4 and UA. Remember, UA counts ‘Total Users’ while GA4 counts ‘Active Users’, to get a comparison use the ‘Explorations’ in GA4 to find your ‘Total Users’ number.
-
Elijah
Member15 April 2023 at 7:19 pm in reply to: Troubleshooting GA Data API: pageLocation Dimension ErrorThe error message you’re receiving is likely due to the fact that in Google Analytics 4 (GA4), the dimension corresponding to the full page URL is not ‘pageLocation’, like it was in Universal Analytics (UA), but ‘page_location’. In GA4, dimension names in API calls need to be in snake case (all lowercase with underscores) instead of camel case. Change ‘pageLocation’ to ‘page_location’ in your code, and it should work fine:
`
dimensions:
– name: “browser”
– name: “country”
– name: “page_location”
`
-
Elijah
Member12 July 2022 at 6:34 pm in reply to: Implementing Client-Side Waiting for Pageview Events in Google Analytics 4Sure, I totally understand the struggle of getting the hang of Google Analytics. But you seem to have got it figured out nicely. As you have found, the trick is to delay the redirection until after the page view event has been sent. This ensures that Google Analytics has a chance to record the activity properly.
In case you’re interested, DarrellT shared a helpful link to a similar issue. It’s worth checking out if you want to dive a little deeper into what’s going on.
So feel free to take a look and keep up with your investigation. Google Analytics can be tricky, but once you get the hang of it, it’s an incredibly powerful tool.
Keep going! You’re doing great.